iced_widget/pane_grid.rs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 485 486 487 488 489 490 491 492 493 494 495 496 497 498 499 500 501 502 503 504 505 506 507 508 509 510 511 512 513 514 515 516 517 518 519 520 521 522 523 524 525 526 527 528 529 530 531 532 533 534 535 536 537 538 539 540 541 542 543 544 545 546 547 548 549 550 551 552 553 554 555 556 557 558 559 560 561 562 563 564 565 566 567 568 569 570 571 572 573 574 575 576 577 578 579 580 581 582 583 584 585 586 587 588 589 590 591 592 593 594 595 596 597 598 599 600 601 602 603 604 605 606 607 608 609 610 611 612 613 614 615 616 617 618 619 620 621 622 623 624 625 626 627 628 629 630 631 632 633 634 635 636 637 638 639 640 641 642 643 644 645 646 647 648 649 650 651 652 653 654 655 656 657 658 659 660 661 662 663 664 665 666 667 668 669 670 671 672 673 674 675 676 677 678 679 680 681 682 683 684 685 686 687 688 689 690 691 692 693 694 695 696 697 698 699 700 701 702 703 704 705 706 707 708 709 710 711 712 713 714 715 716 717 718 719 720 721 722 723 724 725 726 727 728 729 730 731 732 733 734 735 736 737 738 739 740 741 742 743 744 745 746 747 748 749 750 751 752 753 754 755 756 757 758 759 760 761 762 763 764 765 766 767 768 769 770 771 772 773 774 775 776 777 778 779 780 781 782 783 784 785 786 787 788 789 790 791 792 793 794 795 796 797 798 799 800 801 802 803 804 805 806 807 808 809 810 811 812 813 814 815 816 817 818 819 820 821 822 823 824 825 826 827 828 829 830 831 832 833 834 835 836 837 838 839 840 841 842 843 844 845 846 847 848 849 850 851 852 853 854 855 856 857 858 859 860 861 862 863 864 865 866 867 868 869 870 871 872 873 874 875 876 877 878 879 880 881 882 883 884 885 886 887 888 889 890 891 892 893 894 895 896 897 898 899 900 901 902 903 904 905 906 907 908 909 910 911 912 913 914 915 916 917 918 919 920 921 922 923 924 925 926 927 928 929 930 931 932 933 934 935 936 937 938 939 940 941 942 943 944 945 946 947 948 949 950 951 952 953 954 955 956 957 958 959 960 961 962 963 964 965 966 967 968 969 970 971 972 973 974 975 976 977 978 979 980 981 982 983 984 985 986 987 988 989 990 991 992 993 994 995 996 997 998 999 1000 1001 1002 1003 1004 1005 1006 1007 1008 1009 1010 1011 1012 1013 1014 1015 1016 1017 1018 1019 1020 1021 1022 1023 1024 1025 1026 1027 1028 1029 1030 1031 1032 1033 1034 1035 1036 1037 1038 1039 1040 1041 1042 1043 1044 1045 1046 1047 1048 1049 1050 1051 1052 1053 1054 1055 1056 1057 1058 1059 1060 1061 1062 1063 1064 1065 1066 1067 1068 1069 1070 1071 1072 1073 1074 1075 1076 1077 1078 1079 1080 1081 1082 1083 1084 1085 1086 1087 1088 1089 1090 1091 1092 1093 1094 1095 1096 1097 1098 1099 1100 1101 1102 1103 1104 1105 1106 1107 1108 1109 1110 1111 1112 1113 1114 1115 1116 1117 1118 1119 1120 1121 1122 1123 1124 1125 1126 1127 1128 1129 1130 1131 1132 1133 1134 1135 1136 1137 1138 1139 1140 1141 1142 1143 1144 1145 1146 1147 1148 1149 1150 1151 1152 1153 1154 1155 1156 1157 1158 1159 1160 1161 1162 1163 1164 1165 1166 1167 1168 1169 1170 1171 1172 1173 1174 1175 1176 1177 1178 1179 1180 1181 1182 1183 1184 1185 1186 1187 1188 1189 1190 1191 1192 1193 1194 1195 1196 1197 1198 1199 1200 1201 1202 1203 1204 1205 1206 1207 1208 1209 1210 1211 1212 1213 1214 1215 1216 1217 1218 1219 1220 1221 1222 1223 1224 1225 1226 1227 1228 1229 1230 1231 1232 1233 1234 1235 1236 1237 1238 1239 1240 1241 1242 1243 1244 1245 1246 1247 1248 1249 1250 1251 1252 1253 1254 1255 1256 1257 1258 1259 1260 1261 1262 1263 1264 1265 1266 1267 1268 1269 1270 1271 1272 1273 1274 1275 1276 1277 1278 1279 1280 1281 1282 1283 1284 1285 1286 1287 1288 1289 1290 1291 1292 1293 1294 1295 1296 1297 1298 1299 1300 1301 1302 1303 1304 1305 1306 1307 1308 1309 1310 1311 1312 1313 1314 1315 1316 1317 1318 1319 1320 1321 1322 1323 1324 1325 1326 1327 1328 1329 1330 1331 1332 1333 1334 1335 1336 1337 1338 1339 1340
//! Pane grids let your users split regions of your application and organize layout dynamically.
//!
//! 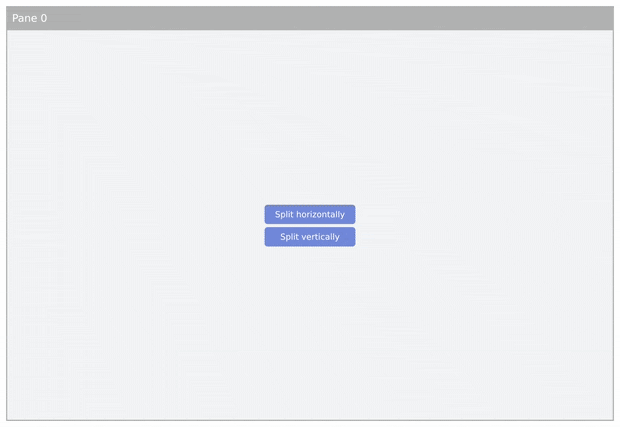
//!
//! This distribution of space is common in tiling window managers (like
//! [`awesome`](https://awesomewm.org/), [`i3`](https://i3wm.org/), or even
//! [`tmux`](https://github.com/tmux/tmux)).
//!
//! A [`PaneGrid`] supports:
//!
//! * Vertical and horizontal splits
//! * Tracking of the last active pane
//! * Mouse-based resizing
//! * Drag and drop to reorganize panes
//! * Hotkey support
//! * Configurable modifier keys
//! * [`State`] API to perform actions programmatically (`split`, `swap`, `resize`, etc.)
//!
//! # Example
//! ```no_run
//! # mod iced { pub mod widget { pub use iced_widget::*; } pub use iced_widget::Renderer; pub use iced_widget::core::*; }
//! # pub type Element<'a, Message> = iced_widget::core::Element<'a, Message, iced_widget::Theme, iced_widget::Renderer>;
//! #
//! use iced::widget::{pane_grid, text};
//!
//! struct State {
//! panes: pane_grid::State<Pane>,
//! }
//!
//! enum Pane {
//! SomePane,
//! AnotherKindOfPane,
//! }
//!
//! enum Message {
//! PaneDragged(pane_grid::DragEvent),
//! PaneResized(pane_grid::ResizeEvent),
//! }
//!
//! fn view(state: &State) -> Element<'_, Message> {
//! pane_grid(&state.panes, |pane, state, is_maximized| {
//! pane_grid::Content::new(match state {
//! Pane::SomePane => text("This is some pane"),
//! Pane::AnotherKindOfPane => text("This is another kind of pane"),
//! })
//! })
//! .on_drag(Message::PaneDragged)
//! .on_resize(10, Message::PaneResized)
//! .into()
//! }
//! ```
//! The [`pane_grid` example] showcases how to use a [`PaneGrid`] with resizing,
//! drag and drop, and hotkey support.
//!
//! [`pane_grid` example]: https://github.com/iced-rs/iced/tree/0.13/examples/pane_grid
mod axis;
mod configuration;
mod content;
mod controls;
mod direction;
mod draggable;
mod node;
mod pane;
mod split;
mod title_bar;
pub mod state;
pub use axis::Axis;
pub use configuration::Configuration;
pub use content::Content;
pub use controls::Controls;
pub use direction::Direction;
pub use draggable::Draggable;
pub use node::Node;
pub use pane::Pane;
pub use split::Split;
pub use state::State;
pub use title_bar::TitleBar;
use crate::container;
use crate::core::layout;
use crate::core::mouse;
use crate::core::overlay::{self, Group};
use crate::core::renderer;
use crate::core::touch;
use crate::core::widget;
use crate::core::widget::tree::{self, Tree};
use crate::core::window;
use crate::core::{
self, Background, Border, Clipboard, Color, Element, Event, Layout, Length,
Pixels, Point, Rectangle, Shell, Size, Theme, Vector, Widget,
};
const DRAG_DEADBAND_DISTANCE: f32 = 10.0;
const THICKNESS_RATIO: f32 = 25.0;
/// A collection of panes distributed using either vertical or horizontal splits
/// to completely fill the space available.
///
/// 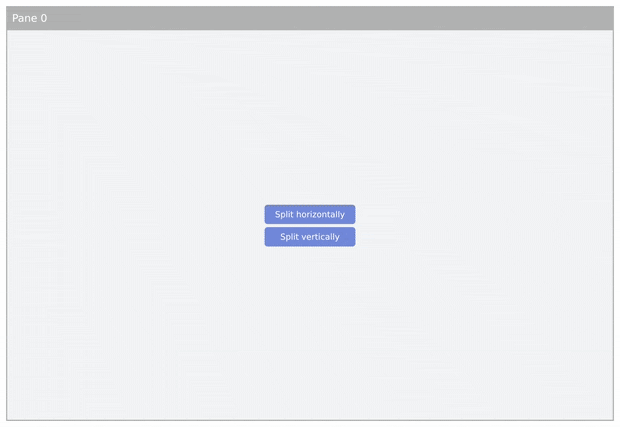
///
/// This distribution of space is common in tiling window managers (like
/// [`awesome`](https://awesomewm.org/), [`i3`](https://i3wm.org/), or even
/// [`tmux`](https://github.com/tmux/tmux)).
///
/// A [`PaneGrid`] supports:
///
/// * Vertical and horizontal splits
/// * Tracking of the last active pane
/// * Mouse-based resizing
/// * Drag and drop to reorganize panes
/// * Hotkey support
/// * Configurable modifier keys
/// * [`State`] API to perform actions programmatically (`split`, `swap`, `resize`, etc.)
///
/// # Example
/// ```no_run
/// # mod iced { pub mod widget { pub use iced_widget::*; } pub use iced_widget::Renderer; pub use iced_widget::core::*; }
/// # pub type Element<'a, Message> = iced_widget::core::Element<'a, Message, iced_widget::Theme, iced_widget::Renderer>;
/// #
/// use iced::widget::{pane_grid, text};
///
/// struct State {
/// panes: pane_grid::State<Pane>,
/// }
///
/// enum Pane {
/// SomePane,
/// AnotherKindOfPane,
/// }
///
/// enum Message {
/// PaneDragged(pane_grid::DragEvent),
/// PaneResized(pane_grid::ResizeEvent),
/// }
///
/// fn view(state: &State) -> Element<'_, Message> {
/// pane_grid(&state.panes, |pane, state, is_maximized| {
/// pane_grid::Content::new(match state {
/// Pane::SomePane => text("This is some pane"),
/// Pane::AnotherKindOfPane => text("This is another kind of pane"),
/// })
/// })
/// .on_drag(Message::PaneDragged)
/// .on_resize(10, Message::PaneResized)
/// .into()
/// }
/// ```
#[allow(missing_debug_implementations)]
pub struct PaneGrid<
'a,
Message,
Theme = crate::Theme,
Renderer = crate::Renderer,
> where
Theme: Catalog,
Renderer: core::Renderer,
{
internal: &'a state::Internal,
panes: Vec<Pane>,
contents: Vec<Content<'a, Message, Theme, Renderer>>,
width: Length,
height: Length,
spacing: f32,
on_click: Option<Box<dyn Fn(Pane) -> Message + 'a>>,
on_drag: Option<Box<dyn Fn(DragEvent) -> Message + 'a>>,
on_resize: Option<(f32, Box<dyn Fn(ResizeEvent) -> Message + 'a>)>,
class: <Theme as Catalog>::Class<'a>,
last_mouse_interaction: Option<mouse::Interaction>,
}
impl<'a, Message, Theme, Renderer> PaneGrid<'a, Message, Theme, Renderer>
where
Theme: Catalog,
Renderer: core::Renderer,
{
/// Creates a [`PaneGrid`] with the given [`State`] and view function.
///
/// The view function will be called to display each [`Pane`] present in the
/// [`State`]. [`bool`] is set if the pane is maximized.
pub fn new<T>(
state: &'a State<T>,
view: impl Fn(Pane, &'a T, bool) -> Content<'a, Message, Theme, Renderer>,
) -> Self {
let panes = state.panes.keys().copied().collect();
let contents = state
.panes
.iter()
.map(|(pane, pane_state)| match state.maximized() {
Some(p) if *pane == p => view(*pane, pane_state, true),
_ => view(*pane, pane_state, false),
})
.collect();
Self {
internal: &state.internal,
panes,
contents,
width: Length::Fill,
height: Length::Fill,
spacing: 0.0,
on_click: None,
on_drag: None,
on_resize: None,
class: <Theme as Catalog>::default(),
last_mouse_interaction: None,
}
}
/// Sets the width of the [`PaneGrid`].
pub fn width(mut self, width: impl Into<Length>) -> Self {
self.width = width.into();
self
}
/// Sets the height of the [`PaneGrid`].
pub fn height(mut self, height: impl Into<Length>) -> Self {
self.height = height.into();
self
}
/// Sets the spacing _between_ the panes of the [`PaneGrid`].
pub fn spacing(mut self, amount: impl Into<Pixels>) -> Self {
self.spacing = amount.into().0;
self
}
/// Sets the message that will be produced when a [`Pane`] of the
/// [`PaneGrid`] is clicked.
pub fn on_click<F>(mut self, f: F) -> Self
where
F: 'a + Fn(Pane) -> Message,
{
self.on_click = Some(Box::new(f));
self
}
/// Enables the drag and drop interactions of the [`PaneGrid`], which will
/// use the provided function to produce messages.
pub fn on_drag<F>(mut self, f: F) -> Self
where
F: 'a + Fn(DragEvent) -> Message,
{
if self.internal.maximized().is_none() {
self.on_drag = Some(Box::new(f));
}
self
}
/// Enables the resize interactions of the [`PaneGrid`], which will
/// use the provided function to produce messages.
///
/// The `leeway` describes the amount of space around a split that can be
/// used to grab it.
///
/// The grabbable area of a split will have a length of `spacing + leeway`,
/// properly centered. In other words, a length of
/// `(spacing + leeway) / 2.0` on either side of the split line.
pub fn on_resize<F>(mut self, leeway: impl Into<Pixels>, f: F) -> Self
where
F: 'a + Fn(ResizeEvent) -> Message,
{
if self.internal.maximized().is_none() {
self.on_resize = Some((leeway.into().0, Box::new(f)));
}
self
}
/// Sets the style of the [`PaneGrid`].
#[must_use]
pub fn style(mut self, style: impl Fn(&Theme) -> Style + 'a) -> Self
where
<Theme as Catalog>::Class<'a>: From<StyleFn<'a, Theme>>,
{
self.class = (Box::new(style) as StyleFn<'a, Theme>).into();
self
}
/// Sets the style class of the [`PaneGrid`].
#[cfg(feature = "advanced")]
#[must_use]
pub fn class(
mut self,
class: impl Into<<Theme as Catalog>::Class<'a>>,
) -> Self {
self.class = class.into();
self
}
fn drag_enabled(&self) -> bool {
self.internal
.maximized()
.is_none()
.then(|| self.on_drag.is_some())
.unwrap_or_default()
}
fn grid_interaction(
&self,
action: &state::Action,
layout: Layout<'_>,
cursor: mouse::Cursor,
) -> Option<mouse::Interaction> {
if action.picked_pane().is_some() {
return Some(mouse::Interaction::Grabbing);
}
let resize_leeway = self.on_resize.as_ref().map(|(leeway, _)| *leeway);
let node = self.internal.layout();
let resize_axis =
action.picked_split().map(|(_, axis)| axis).or_else(|| {
resize_leeway.and_then(|leeway| {
let cursor_position = cursor.position()?;
let bounds = layout.bounds();
let splits =
node.split_regions(self.spacing, bounds.size());
let relative_cursor = Point::new(
cursor_position.x - bounds.x,
cursor_position.y - bounds.y,
);
hovered_split(
splits.iter(),
self.spacing + leeway,
relative_cursor,
)
.map(|(_, axis, _)| axis)
})
});
if let Some(resize_axis) = resize_axis {
return Some(match resize_axis {
Axis::Horizontal => mouse::Interaction::ResizingVertically,
Axis::Vertical => mouse::Interaction::ResizingHorizontally,
});
}
None
}
}
#[derive(Default)]
struct Memory {
action: state::Action,
order: Vec<Pane>,
}
impl<Message, Theme, Renderer> Widget<Message, Theme, Renderer>
for PaneGrid<'_, Message, Theme, Renderer>
where
Theme: Catalog,
Renderer: core::Renderer,
{
fn tag(&self) -> tree::Tag {
tree::Tag::of::<Memory>()
}
fn state(&self) -> tree::State {
tree::State::new(Memory::default())
}
fn children(&self) -> Vec<Tree> {
self.contents.iter().map(Content::state).collect()
}
fn diff(&self, tree: &mut Tree) {
let Memory { order, .. } = tree.state.downcast_ref();
// `Pane` always increments and is iterated by Ord so new
// states are always added at the end. We can simply remove
// states which no longer exist and `diff_children` will
// diff the remaining values in the correct order and
// add new states at the end
let mut i = 0;
let mut j = 0;
tree.children.retain(|_| {
let retain = self.panes.get(i) == order.get(j);
if retain {
i += 1;
}
j += 1;
retain
});
tree.diff_children_custom(
&self.contents,
|state, content| content.diff(state),
Content::state,
);
let Memory { order, .. } = tree.state.downcast_mut();
order.clone_from(&self.panes);
}
fn size(&self) -> Size<Length> {
Size {
width: self.width,
height: self.height,
}
}
fn layout(
&self,
tree: &mut Tree,
renderer: &Renderer,
limits: &layout::Limits,
) -> layout::Node {
let size = limits.resolve(self.width, self.height, Size::ZERO);
let regions = self.internal.layout().pane_regions(self.spacing, size);
let children = self
.panes
.iter()
.copied()
.zip(&self.contents)
.zip(tree.children.iter_mut())
.filter_map(|((pane, content), tree)| {
if self
.internal
.maximized()
.is_some_and(|maximized| maximized != pane)
{
return Some(layout::Node::new(Size::ZERO));
}
let region = regions.get(&pane)?;
let size = Size::new(region.width, region.height);
let node = content.layout(
tree,
renderer,
&layout::Limits::new(size, size),
);
Some(node.move_to(Point::new(region.x, region.y)))
})
.collect();
layout::Node::with_children(size, children)
}
fn operate(
&self,
tree: &mut Tree,
layout: Layout<'_>,
renderer: &Renderer,
operation: &mut dyn widget::Operation,
) {
operation.container(None, layout.bounds(), &mut |operation| {
self.panes
.iter()
.copied()
.zip(&self.contents)
.zip(&mut tree.children)
.zip(layout.children())
.filter(|(((pane, _), _), _)| {
self.internal
.maximized()
.map_or(true, |maximized| *pane == maximized)
})
.for_each(|(((_, content), state), layout)| {
content.operate(state, layout, renderer, operation);
});
});
}
fn update(
&mut self,
tree: &mut Tree,
event: Event,
layout: Layout<'_>,
cursor: mouse::Cursor,
renderer: &Renderer,
clipboard: &mut dyn Clipboard,
shell: &mut Shell<'_, Message>,
viewport: &Rectangle,
) {
let Memory { action, .. } = tree.state.downcast_mut();
let node = self.internal.layout();
let on_drag = if self.drag_enabled() {
&self.on_drag
} else {
&None
};
let picked_pane = action.picked_pane().map(|(pane, _)| pane);
for (((pane, content), tree), layout) in self
.panes
.iter()
.copied()
.zip(&mut self.contents)
.zip(&mut tree.children)
.zip(layout.children())
.filter(|(((pane, _), _), _)| {
self.internal
.maximized()
.map_or(true, |maximized| *pane == maximized)
})
{
let is_picked = picked_pane == Some(pane);
content.update(
tree,
event.clone(),
layout,
cursor,
renderer,
clipboard,
shell,
viewport,
is_picked,
);
}
match event {
Event::Mouse(mouse::Event::ButtonPressed(mouse::Button::Left))
| Event::Touch(touch::Event::FingerPressed { .. }) => {
let bounds = layout.bounds();
if let Some(cursor_position) = cursor.position_over(bounds) {
shell.capture_event();
match &self.on_resize {
Some((leeway, _)) => {
let relative_cursor = Point::new(
cursor_position.x - bounds.x,
cursor_position.y - bounds.y,
);
let splits = node.split_regions(
self.spacing,
Size::new(bounds.width, bounds.height),
);
let clicked_split = hovered_split(
splits.iter(),
self.spacing + leeway,
relative_cursor,
);
if let Some((split, axis, _)) = clicked_split {
if action.picked_pane().is_none() {
*action =
state::Action::Resizing { split, axis };
}
} else {
click_pane(
action,
layout,
cursor_position,
shell,
self.panes
.iter()
.copied()
.zip(&self.contents),
&self.on_click,
on_drag,
);
}
}
None => {
click_pane(
action,
layout,
cursor_position,
shell,
self.panes.iter().copied().zip(&self.contents),
&self.on_click,
on_drag,
);
}
}
}
}
Event::Mouse(mouse::Event::ButtonReleased(mouse::Button::Left))
| Event::Touch(touch::Event::FingerLifted { .. })
| Event::Touch(touch::Event::FingerLost { .. }) => {
if let Some((pane, origin)) = action.picked_pane() {
if let Some(on_drag) = on_drag {
if let Some(cursor_position) = cursor.position() {
if cursor_position.distance(origin)
> DRAG_DEADBAND_DISTANCE
{
let event = if let Some(edge) =
in_edge(layout, cursor_position)
{
DragEvent::Dropped {
pane,
target: Target::Edge(edge),
}
} else {
let dropped_region = self
.panes
.iter()
.copied()
.zip(&self.contents)
.zip(layout.children())
.find_map(|(target, layout)| {
layout_region(
layout,
cursor_position,
)
.map(|region| (target, region))
});
match dropped_region {
Some(((target, _), region))
if pane != target =>
{
DragEvent::Dropped {
pane,
target: Target::Pane(
target, region,
),
}
}
_ => DragEvent::Canceled { pane },
}
};
shell.publish(on_drag(event));
} else {
shell.publish(on_drag(DragEvent::Canceled {
pane,
}));
}
}
}
}
*action = state::Action::Idle;
}
Event::Mouse(mouse::Event::CursorMoved { .. })
| Event::Touch(touch::Event::FingerMoved { .. }) => {
if let Some((_, on_resize)) = &self.on_resize {
if let Some((split, _)) = action.picked_split() {
let bounds = layout.bounds();
let splits = node.split_regions(
self.spacing,
Size::new(bounds.width, bounds.height),
);
if let Some((axis, rectangle, _)) = splits.get(&split) {
if let Some(cursor_position) = cursor.position() {
let ratio = match axis {
Axis::Horizontal => {
let position = cursor_position.y
- bounds.y
- rectangle.y;
(position / rectangle.height)
.clamp(0.1, 0.9)
}
Axis::Vertical => {
let position = cursor_position.x
- bounds.x
- rectangle.x;
(position / rectangle.width)
.clamp(0.1, 0.9)
}
};
shell.publish(on_resize(ResizeEvent {
split,
ratio,
}));
shell.capture_event();
}
}
} else if action.picked_pane().is_some() {
shell.request_redraw();
}
}
}
_ => {}
}
if shell.redraw_request() != Some(window::RedrawRequest::NextFrame) {
let interaction = self
.grid_interaction(action, layout, cursor)
.or_else(|| {
self.panes
.iter()
.zip(&self.contents)
.zip(layout.children())
.filter(|((&pane, _content), _layout)| {
self.internal
.maximized()
.map_or(true, |maximized| pane == maximized)
})
.find_map(|((_pane, content), layout)| {
content.grid_interaction(
layout,
cursor,
on_drag.is_some(),
)
})
})
.unwrap_or(mouse::Interaction::None);
if let Event::Window(window::Event::RedrawRequested(_now)) = event {
self.last_mouse_interaction = Some(interaction);
} else if self.last_mouse_interaction.is_some_and(
|last_mouse_interaction| last_mouse_interaction != interaction,
) {
shell.request_redraw();
}
}
}
fn mouse_interaction(
&self,
tree: &Tree,
layout: Layout<'_>,
cursor: mouse::Cursor,
viewport: &Rectangle,
renderer: &Renderer,
) -> mouse::Interaction {
let Memory { action, .. } = tree.state.downcast_ref();
if let Some(grid_interaction) =
self.grid_interaction(action, layout, cursor)
{
return grid_interaction;
}
self.panes
.iter()
.copied()
.zip(&self.contents)
.zip(&tree.children)
.zip(layout.children())
.filter(|(((pane, _), _), _)| {
self.internal
.maximized()
.map_or(true, |maximized| *pane == maximized)
})
.map(|(((_, content), tree), layout)| {
content.mouse_interaction(
tree,
layout,
cursor,
viewport,
renderer,
self.drag_enabled(),
)
})
.max()
.unwrap_or_default()
}
fn draw(
&self,
tree: &Tree,
renderer: &mut Renderer,
theme: &Theme,
defaults: &renderer::Style,
layout: Layout<'_>,
cursor: mouse::Cursor,
viewport: &Rectangle,
) {
let Memory { action, .. } = tree.state.downcast_ref();
let node = self.internal.layout();
let resize_leeway = self.on_resize.as_ref().map(|(leeway, _)| *leeway);
let picked_pane = action.picked_pane().filter(|(_, origin)| {
cursor
.position()
.map(|position| position.distance(*origin))
.unwrap_or_default()
> DRAG_DEADBAND_DISTANCE
});
let picked_split = action
.picked_split()
.and_then(|(split, axis)| {
let bounds = layout.bounds();
let splits = node.split_regions(self.spacing, bounds.size());
let (_axis, region, ratio) = splits.get(&split)?;
let region =
axis.split_line_bounds(*region, *ratio, self.spacing);
Some((axis, region + Vector::new(bounds.x, bounds.y), true))
})
.or_else(|| match resize_leeway {
Some(leeway) => {
let cursor_position = cursor.position()?;
let bounds = layout.bounds();
let relative_cursor = Point::new(
cursor_position.x - bounds.x,
cursor_position.y - bounds.y,
);
let splits =
node.split_regions(self.spacing, bounds.size());
let (_split, axis, region) = hovered_split(
splits.iter(),
self.spacing + leeway,
relative_cursor,
)?;
Some((
axis,
region + Vector::new(bounds.x, bounds.y),
false,
))
}
None => None,
});
let pane_cursor = if picked_pane.is_some() {
mouse::Cursor::Unavailable
} else {
cursor
};
let mut render_picked_pane = None;
let pane_in_edge = if picked_pane.is_some() {
cursor
.position()
.and_then(|cursor_position| in_edge(layout, cursor_position))
} else {
None
};
let style = Catalog::style(theme, &self.class);
for (((id, content), tree), pane_layout) in self
.panes
.iter()
.copied()
.zip(&self.contents)
.zip(&tree.children)
.zip(layout.children())
.filter(|(((pane, _), _), _)| {
self.internal
.maximized()
.map_or(true, |maximized| maximized == *pane)
})
{
match picked_pane {
Some((dragging, origin)) if id == dragging => {
render_picked_pane =
Some(((content, tree), origin, pane_layout));
}
Some((dragging, _)) if id != dragging => {
content.draw(
tree,
renderer,
theme,
defaults,
pane_layout,
pane_cursor,
viewport,
);
if picked_pane.is_some() && pane_in_edge.is_none() {
if let Some(region) =
cursor.position().and_then(|cursor_position| {
layout_region(pane_layout, cursor_position)
})
{
let bounds =
layout_region_bounds(pane_layout, region);
renderer.fill_quad(
renderer::Quad {
bounds,
border: style.hovered_region.border,
..renderer::Quad::default()
},
style.hovered_region.background,
);
}
}
}
_ => {
content.draw(
tree,
renderer,
theme,
defaults,
pane_layout,
pane_cursor,
viewport,
);
}
}
}
if let Some(edge) = pane_in_edge {
let bounds = edge_bounds(layout, edge);
renderer.fill_quad(
renderer::Quad {
bounds,
border: style.hovered_region.border,
..renderer::Quad::default()
},
style.hovered_region.background,
);
}
// Render picked pane last
if let Some(((content, tree), origin, layout)) = render_picked_pane {
if let Some(cursor_position) = cursor.position() {
let bounds = layout.bounds();
let translation =
cursor_position - Point::new(origin.x, origin.y);
renderer.with_translation(translation, |renderer| {
renderer.with_layer(bounds, |renderer| {
content.draw(
tree,
renderer,
theme,
defaults,
layout,
pane_cursor,
viewport,
);
});
});
}
}
if picked_pane.is_none() {
if let Some((axis, split_region, is_picked)) = picked_split {
let highlight = if is_picked {
style.picked_split
} else {
style.hovered_split
};
renderer.fill_quad(
renderer::Quad {
bounds: match axis {
Axis::Horizontal => Rectangle {
x: split_region.x,
y: (split_region.y
+ (split_region.height - highlight.width)
/ 2.0)
.round(),
width: split_region.width,
height: highlight.width,
},
Axis::Vertical => Rectangle {
x: (split_region.x
+ (split_region.width - highlight.width)
/ 2.0)
.round(),
y: split_region.y,
width: highlight.width,
height: split_region.height,
},
},
..renderer::Quad::default()
},
highlight.color,
);
}
}
}
fn overlay<'b>(
&'b mut self,
tree: &'b mut Tree,
layout: Layout<'_>,
renderer: &Renderer,
translation: Vector,
) -> Option<overlay::Element<'b, Message, Theme, Renderer>> {
let children = self
.panes
.iter()
.copied()
.zip(&mut self.contents)
.zip(&mut tree.children)
.zip(layout.children())
.filter_map(|(((pane, content), state), layout)| {
if self
.internal
.maximized()
.is_some_and(|maximized| maximized != pane)
{
return None;
}
content.overlay(state, layout, renderer, translation)
})
.collect::<Vec<_>>();
(!children.is_empty()).then(|| Group::with_children(children).overlay())
}
}
impl<'a, Message, Theme, Renderer> From<PaneGrid<'a, Message, Theme, Renderer>>
for Element<'a, Message, Theme, Renderer>
where
Message: 'a,
Theme: Catalog + 'a,
Renderer: core::Renderer + 'a,
{
fn from(
pane_grid: PaneGrid<'a, Message, Theme, Renderer>,
) -> Element<'a, Message, Theme, Renderer> {
Element::new(pane_grid)
}
}
fn layout_region(layout: Layout<'_>, cursor_position: Point) -> Option<Region> {
let bounds = layout.bounds();
if !bounds.contains(cursor_position) {
return None;
}
let region = if cursor_position.x < (bounds.x + bounds.width / 3.0) {
Region::Edge(Edge::Left)
} else if cursor_position.x > (bounds.x + 2.0 * bounds.width / 3.0) {
Region::Edge(Edge::Right)
} else if cursor_position.y < (bounds.y + bounds.height / 3.0) {
Region::Edge(Edge::Top)
} else if cursor_position.y > (bounds.y + 2.0 * bounds.height / 3.0) {
Region::Edge(Edge::Bottom)
} else {
Region::Center
};
Some(region)
}
fn click_pane<'a, Message, T>(
action: &mut state::Action,
layout: Layout<'_>,
cursor_position: Point,
shell: &mut Shell<'_, Message>,
contents: impl Iterator<Item = (Pane, T)>,
on_click: &Option<Box<dyn Fn(Pane) -> Message + 'a>>,
on_drag: &Option<Box<dyn Fn(DragEvent) -> Message + 'a>>,
) where
T: Draggable,
{
let mut clicked_region = contents
.zip(layout.children())
.filter(|(_, layout)| layout.bounds().contains(cursor_position));
if let Some(((pane, content), layout)) = clicked_region.next() {
if let Some(on_click) = &on_click {
shell.publish(on_click(pane));
}
if let Some(on_drag) = &on_drag {
if content.can_be_dragged_at(layout, cursor_position) {
*action = state::Action::Dragging {
pane,
origin: cursor_position,
};
shell.publish(on_drag(DragEvent::Picked { pane }));
}
}
}
}
fn in_edge(layout: Layout<'_>, cursor: Point) -> Option<Edge> {
let bounds = layout.bounds();
let height_thickness = bounds.height / THICKNESS_RATIO;
let width_thickness = bounds.width / THICKNESS_RATIO;
let thickness = height_thickness.min(width_thickness);
if cursor.x > bounds.x && cursor.x < bounds.x + thickness {
Some(Edge::Left)
} else if cursor.x > bounds.x + bounds.width - thickness
&& cursor.x < bounds.x + bounds.width
{
Some(Edge::Right)
} else if cursor.y > bounds.y && cursor.y < bounds.y + thickness {
Some(Edge::Top)
} else if cursor.y > bounds.y + bounds.height - thickness
&& cursor.y < bounds.y + bounds.height
{
Some(Edge::Bottom)
} else {
None
}
}
fn edge_bounds(layout: Layout<'_>, edge: Edge) -> Rectangle {
let bounds = layout.bounds();
let height_thickness = bounds.height / THICKNESS_RATIO;
let width_thickness = bounds.width / THICKNESS_RATIO;
let thickness = height_thickness.min(width_thickness);
match edge {
Edge::Top => Rectangle {
height: thickness,
..bounds
},
Edge::Left => Rectangle {
width: thickness,
..bounds
},
Edge::Right => Rectangle {
x: bounds.x + bounds.width - thickness,
width: thickness,
..bounds
},
Edge::Bottom => Rectangle {
y: bounds.y + bounds.height - thickness,
height: thickness,
..bounds
},
}
}
fn layout_region_bounds(layout: Layout<'_>, region: Region) -> Rectangle {
let bounds = layout.bounds();
match region {
Region::Center => bounds,
Region::Edge(edge) => match edge {
Edge::Top => Rectangle {
height: bounds.height / 2.0,
..bounds
},
Edge::Left => Rectangle {
width: bounds.width / 2.0,
..bounds
},
Edge::Right => Rectangle {
x: bounds.x + bounds.width / 2.0,
width: bounds.width / 2.0,
..bounds
},
Edge::Bottom => Rectangle {
y: bounds.y + bounds.height / 2.0,
height: bounds.height / 2.0,
..bounds
},
},
}
}
/// An event produced during a drag and drop interaction of a [`PaneGrid`].
#[derive(Debug, Clone, Copy)]
pub enum DragEvent {
/// A [`Pane`] was picked for dragging.
Picked {
/// The picked [`Pane`].
pane: Pane,
},
/// A [`Pane`] was dropped on top of another [`Pane`].
Dropped {
/// The picked [`Pane`].
pane: Pane,
/// The [`Target`] where the picked [`Pane`] was dropped on.
target: Target,
},
/// A [`Pane`] was picked and then dropped outside of other [`Pane`]
/// boundaries.
Canceled {
/// The picked [`Pane`].
pane: Pane,
},
}
/// The [`Target`] area a pane can be dropped on.
#[derive(Debug, Clone, Copy)]
pub enum Target {
/// An [`Edge`] of the full [`PaneGrid`].
Edge(Edge),
/// A single [`Pane`] of the [`PaneGrid`].
Pane(Pane, Region),
}
/// The region of a [`Pane`].
#[derive(Debug, Clone, Copy, Default)]
pub enum Region {
/// Center region.
#[default]
Center,
/// Edge region.
Edge(Edge),
}
/// The edges of an area.
#[derive(Debug, Clone, Copy)]
pub enum Edge {
/// Top edge.
Top,
/// Left edge.
Left,
/// Right edge.
Right,
/// Bottom edge.
Bottom,
}
/// An event produced during a resize interaction of a [`PaneGrid`].
#[derive(Debug, Clone, Copy)]
pub struct ResizeEvent {
/// The [`Split`] that is being dragged for resizing.
pub split: Split,
/// The new ratio of the [`Split`].
///
/// The ratio is a value in [0, 1], representing the exact position of a
/// [`Split`] between two panes.
pub ratio: f32,
}
/*
* Helpers
*/
fn hovered_split<'a>(
mut splits: impl Iterator<Item = (&'a Split, &'a (Axis, Rectangle, f32))>,
spacing: f32,
cursor_position: Point,
) -> Option<(Split, Axis, Rectangle)> {
splits.find_map(|(split, (axis, region, ratio))| {
let bounds = axis.split_line_bounds(*region, *ratio, spacing);
if bounds.contains(cursor_position) {
Some((*split, *axis, bounds))
} else {
None
}
})
}
/// The appearance of a [`PaneGrid`].
#[derive(Debug, Clone, Copy, PartialEq)]
pub struct Style {
/// The appearance of a hovered region highlight.
pub hovered_region: Highlight,
/// The appearance of a picked split.
pub picked_split: Line,
/// The appearance of a hovered split.
pub hovered_split: Line,
}
/// The appearance of a highlight of the [`PaneGrid`].
#[derive(Debug, Clone, Copy, PartialEq)]
pub struct Highlight {
/// The [`Background`] of the pane region.
pub background: Background,
/// The [`Border`] of the pane region.
pub border: Border,
}
/// A line.
///
/// It is normally used to define the highlight of something, like a split.
#[derive(Debug, Clone, Copy, PartialEq)]
pub struct Line {
/// The [`Color`] of the [`Line`].
pub color: Color,
/// The width of the [`Line`].
pub width: f32,
}
/// The theme catalog of a [`PaneGrid`].
pub trait Catalog: container::Catalog {
/// The item class of this [`Catalog`].
type Class<'a>;
/// The default class produced by this [`Catalog`].
fn default<'a>() -> <Self as Catalog>::Class<'a>;
/// The [`Style`] of a class with the given status.
fn style(&self, class: &<Self as Catalog>::Class<'_>) -> Style;
}
/// A styling function for a [`PaneGrid`].
///
/// This is just a boxed closure: `Fn(&Theme, Status) -> Style`.
pub type StyleFn<'a, Theme> = Box<dyn Fn(&Theme) -> Style + 'a>;
impl Catalog for Theme {
type Class<'a> = StyleFn<'a, Self>;
fn default<'a>() -> StyleFn<'a, Self> {
Box::new(default)
}
fn style(&self, class: &StyleFn<'_, Self>) -> Style {
class(self)
}
}
/// The default style of a [`PaneGrid`].
pub fn default(theme: &Theme) -> Style {
let palette = theme.extended_palette();
Style {
hovered_region: Highlight {
background: Background::Color(Color {
a: 0.5,
..palette.primary.base.color
}),
border: Border {
width: 2.0,
color: palette.primary.strong.color,
radius: 0.0.into(),
},
},
hovered_split: Line {
color: palette.primary.base.color,
width: 2.0,
},
picked_split: Line {
color: palette.primary.strong.color,
width: 2.0,
},
}
}